- Square Star Pattern
- Half Pyramid star Pattern
- Inverted half Pyramid -1
- Inverted half Pyramid – 2
- Inverted half Pyramid – 3
- Inverted half Pyramid – 4
- Half Pyramid Number – 1
- Half Pyramid Number – 2
- Inverted Half Pyramid
- Triangle 0/1 star
- Diamond Star Pattern
- Half Diamond star
- Reverse Pyramid star-1
- Reverse Pyramid star-2
- Hellow Rectangle star
- Character Triangle – 1
- Character triangle -2
- Heart Star Pattern
Introduction To Java
- java is a class based , high – level , object oriented programming language.
- Developed by “james gosling and his friends in the year – 1995.
- The first version of java (JDK-1.0) was released on the year – janvary 23rd 1996 by “sun microsystem”.
- Java Symbol
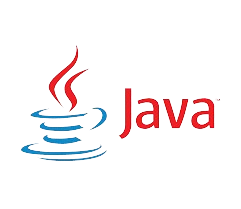
Application of Java
- Mobile Application ( Android Apps)
- Desktop Application
- Gaming Application
- Big Data technologies
- Business Applications
- Web servers and application servers
- Web applications
- Enterprise Applications
- Database connection
- Scientific Applications
About Java History
- Java is a totally computer based programming language developed by sun microsystem ( james gosling , mike sheridon & patric Naughton).
- In the year 1991 james gosling & his friend start a project.
- Team = Green team
- James gosling = greentalk (first name)
- Extension = .gt
- Greentalk = oak ( second name )
- Oak change name region 1. National tree(u.s.a , france etc.) 2. Oak technology company name.
- Oak = java(1995)
- Java = setbox , television , remote ect..
- Java = internet programming
- Version = JDK alpha & Beta , 1995 ( By sum microsystem).
- Sun microsystem = oracle corporation ( 2010 ).
- Java – 1. Core java 2. Advance java 3. Android java
Features Of Java
- Object oriented
- Platform independent
- Simple and easy to learn
- Open sorce
- Secure
- Portable
- Compiled & interpreted
- Robust language
- Distributed
- Large Library & frameworks
- Multi-threaded
- Performance
- Dynamic
JDK JRE JVM ?
JDK= JDK stands for java development kit , it internally contains JRE & JVM where JRE stands for Java Runtime Environment and JVM stands for Java Virtual Machine. JDK provides all tools to work with java language.
2. JRE= JRE stands for java runtime environment , its provide on environment to internally contains JVM which is responsible to execute a java program.
3. JVM= JVM stands for java virtual machine , it is
the software in the form of interpreter writer in ‘C’ language through which we
can execute our java program.
Syntax of Java
{
public static void main(String[]agrs)
{
System.out.print(” “);
}
}
Java Save Process
- Save = class name.java
- Compilation = javac class-name.java
- Execution = java class-name
Java Demo Program
class First
{
public static void main(String[]agrs)
{
System.out.print(“Pushpad Computer Institute”);
}
}
Java Comments
Defination = comments are the statement that are totally ignored by the compiler & interpreter.
Why we use comment:-
- The comments can be used to provide explanation about the program.
- Comments makes program more understandable and redouble to the others.
Types:-
- Single line comment //—————
- Multi line comment /*—————*/
Example:-
/* java Single Line Comment */
class Pushpad
{
public static void main(String[] args)
{
// This is a comment
System.out.println(“Hello World”);
}
}
/* java Multi Line Comment */
class Demo
{
public static void main(String[] args)
{
/* welcome to back pushpad computer institute
First Program Multi line comment */
System.out.println(“Hello World”);
}
}
Q. what is variable ? And types ?
Defination = variable is the name of memory location. Where we stored different types of value. It is user difind name which is given by user.
Types of variable :-
- Local = { ——– } (andar likha ho )
- Static = static keyword laga hota hai.
- Instance = class {——{—-}———} //global variable
- Final = his not value change.
- Local variable = A variable which is declared inside the body of the method or method parameter called local variable.
void fun (int a)
{
int x; //local variable
}
2. Instance variable :- a variable which is declared inside the class but outside of all the methods called instance variables.
Syntax =
Class A
{
int a; //instance variable
public static void main(string[]agrs)
{
//code
}
}
1. Static variable :- a variable which is declared with the help of static keyword called static variable.
Syntax=
static int x;
class A
{
static int m=100;//static variable
void method()
{
int n=90;//local variable
}
public static void main(String args[])
{
int data=50;//instance variable
}
}//end of class
Q. Defind Rules of Variable declaration.
Rules of Variable declaration:-
i.The name of the variable is the combination of characters ( A to Z or a to z ) , numbers ( 0 to 9 ) and two special symbol such as underscore( _ ) and dollar ( $ ) .
Ex. à int a; int _a; int $a; int a2;
ii. Variable name always mention in the left hand side of assignment operator.
Ex. à int a = 10;
iii. First character of variable name must be a letters or underscore or dollar.
Ex. à int a; int _a; int $a;
iv. Their should be no blanks between the name of the variable.
Ex. à S um=10; ( X ) sum=10; ☑
v. Except underscore( _ ) we can’t use any special symbol.in the middle of variable.
Ex. à int su_m=10;
vi. Variable are case – sensitive in java.
Ex. à int AB=10; = S.O.P(abAB);
vii. You can’t use any keywords as a variable name.
Ex. à int int=10; ( X ) , sum=10; ☑
Syntax:- datatype var-name = value;
Int =10;
Q.1 Write a program variable ?
/* Rules of variable Declaration */
class A {
public static void main(String[] args) {
int a = 10;
System.out.println(a);
int _b = 20;
System.out.println(_b);
int $c = 30;
System.out.println($c);
int a2 = 40;
System.out.println(a2);
int su_m = 50;
System.out.println(su_m);
int su2m = 60;
System.out.println(su2m);
int AB=100;
System.out.println(AB);
//System.out.println(ab); wrong
// int 2a; –> wrong name
// int su m –> wrong name
// int 10=a; –> wrong name
// int int=105; –> wrong name
}
}
Output:-
10
20
30
40
50
60
100
Q.2 Write a program variable ?
class A
{
static int b = 20; // static variable
int c = 30; // instance variable
public static void main(String[] agrs)
{
int a = 10; // lacal variable
A ref = new A();
System.out.println(a);
System.out.println(A.b);
System.out.println(ref.c);
}
}
Output:- 10
20
30
Java Type Casting
Defination = converting one datatype to another datatype is called typecasting.
Types:-
- Implicit
- Explicit
Implicit typecasting:-
it is automatically performed by the compiler.
Example Program:-
/*Implicit TypeCasting */
class A {
public static void main(String[] args) {
int a = 10; // 4byte
double b = a; // 8byte
System.out.print(b);
}
}
Output:-
10.00
2.Explicit Typecasting = By default the compiler , doesn’t allow the explicit type casting.
/*Explicit TypeCasting */
class A
{
public static void main(String[] args)
{
double a = 10; // 4byte
int b = (int) a; // 8byte
System.out.print(b);
}
}
Output:- 10
Output ( print )
output:- System.out.print();
It is an output statement in java through which we can print the variable , expressions and many more content.
System
- pre-defind class
- java.lang package
out:- it is static member.
In:- it is static member.
print:- pre-defind class.
Input ( Scanner )
Defination = input(Scanner c lass):- scanner is a pre-defined class in java. Which is available in java.util.package.
It is used to get user input.
Syntax:-
Scanner object=new Scanner(System.in);
Rules:-
- If we use scanner class , must hava to create object of scanner class.
- Scanner class method:-
- nextInt(); //intergs
- nextLine(); //string
- nextFloat(); //floating
- nextBoolean(); //true or false
- nextDouble(): //double
- import Scanner class package at the top line of program.
Syntax:- import.java.util.Scanner;
- wrong input(Input int switch Exception)
Introduction To Keywords
A Java keyword is one of 50 reserved terms that have a special function and a set definition in the Java programming language. The fact that the terms are reserved means that they cannot be used as identifiers for any other program elements, including classes, subclasses, variables, methods and objects.
Keywords List
Abstract | continue | for | new | switch |
Assert | default | goto | package | synchronized |
Boolean | do | if | private | this |
Break | double | implements | protected | throw |
Byte | else | import | public | throws |
Case | enum | instanceof | return | transient |
Catch | extends | int | short | try |
Char | final | interface | static | void |
Class | finally | long | strictfp | volatile |
Const | float | native | super | While |
Q. Write a Program to Keyword ?
class A {
public static void main(String[] args) {
int a = 12, b = 23;
System.out.println(“add=” + (a + b));
System.out.println(“sub=” + (a – b));
System.out.println(“mult=” + (a * b));
}
}
Output:-
add=35
sub=-11
mult=276
Q. what is identifier ? Full Explain
Ans :- In java , an identifier is the name of a variable , method , class , package , or interface that is used for the purpose of identification.
Note:-
- keyword can’t be used as a identifier.
- Identifier are case – sensitive.
- We can’t use while space in between identifier.
- Identifier always starts with latter , $ , and (_).
- Class , variable , method , package , void Identifier
- Identifier is user defind name.
Q. Write a Program to identifier ?
import java.util.Scanner;
class First {
public static void main(String[] agrs) {
Scanner r = new Scanner(System.in);
int a, b, c, d, e, f;
System.out.println(“Enter
two value for add=”);
a = r.nextInt();
b = r.nextInt();
System.out.println(“sum=” + (a + b));
System.out.println(“Enter
two value for sub=”);
c = r.nextInt();
d = r.nextInt();
System.out.println(“sub=” + (c – d));
System.out.println(“Enter
two value for multi=”);
e = r.nextInt();
f = r.nextInt();
System.out.println(“multi=” + (e * f));
}
}
Output:-
Enter two value for add=
10
15
sub=25
Enter two value for sub=
30
20
sub=10
Enter two value for multi=
10
10
multi=100
Q.1 write a program two value of sum , substract , multiply and
divide ?
import java.util.Scanner;
class First {
public static void main(String[] agrs) {
Scanner r = new Scanner(System.in);
int a, b, c, d, e, f;
float g, h;
System.out.println(“Enter two value for add=”);
a = r.nextInt();
b = r.nextInt();
System.out.println(“Enter two value for sub=”);
c = r.nextInt();
d = r.nextInt();
System.out.println(“Enter two value for multi=”);
e = r.nextInt();
f = r.nextInt();
System.out.println(“Enter two value for div=”);
g = r.nextFloat();
h = r.nextFloat();
System.out.println(“add=” + (a + b));
System.out.println(“sub=” + (c – d));
System.out.println(“multi=” + (e * f));
System.out.println(“div=” + (g / h));
}
}
Output:-
Enter two value for add=
12
15
Enter two value for sub=
20
30
Enter two value for multi=
10
15
Enter two value for div=
100
10
add=27
sub=-10
multi=150
div=10.0
Q.1 write a program to find area of square ?
/* Area of Square Program */
//area = side*side
import java.util.Scanner;
class Square
{
public static void main(String[] ars)
{
int side, area;
System.out.print(“Enter Square one side lengh = “);
Scanner f = new Scanner(System.in);
side = f.nextInt();
area = side * side;
System.out.print(“Area of Square = ” + area);
}
}
Enter Square one side lengh = 10
Area of Square = 100
Q.2 write a program to find area of Triangle ?
/* Area of Triangle Program */
// input a b c
// 1. find semiperimeter
// 2. area of triangle
import java.util.Scanner;
//import java.lang.Math;
class Triangle
{
public static void main(String[] args)
{
int a, b, c, sp;
double area;
System.out.print(“Enter value for sides of Triangle = “);
Scanner r = new Scanner(System.in);
a = r.nextInt();
b = r.nextInt();
c = r.nextInt();
sp = (a + b + c) / 2;
area = Math.sqrt(sp * (sp – a) * (sp – b) * (sp – c));
System.out.print(“Area of Triangle = ” + area);
}
}
Output:-
Enter value for sides of Triangle = 12
16
20
Area of Triangle = 96.0
Q.3 write a program to find area of Rectangle ?
/* Area of Rectangle Progra */
//formule = input lengh * input breadth
import java.util.Scanner ;
class Rectangle {
public static void main(String[] args) {
int l, b, area;
System.out.print(“Enter lengh and breadth = “);
Scanner r = new Scanner(System.in);
l = r.nextInt();
b = r.nextInt();
area = l * b;
System.out.print(“Area of Rectangle = ” + area);
}
}
output:-
Enter lengh
and breadth = 10 20
Area of Rectangle = 200
Q.4 write a program to find area of circle ?
/* Area of circle */
//formula = PI * r * r
// PI = 22/7 or 3.14
import java.util.Scanner;
class circle {
public static void main(String[] args) {
final double PI = 3.14, area;
int r;
System.out.print(“Enter circle radius = “);
Scanner ref = new Scanner(System.in);
r = ref.nextInt();
area = r * r * PI;
System.out.print(“Area of Circle = “ + area);
}
}
Output:-
Enter circle radius = 10
Area of Circle = 314.0
Q.5 write a program to swap -1 ?
import java.util.Scanner;
class Swap {
public static void main(String[] args) {
int a, b, temp;
System.out.print(“Enter Two Number = “);
Scanner r = new Scanner(System.in);
a = r.nextInt();
b = r.nextInt();
System.out.println(“before Number = “ + a + ” “ + b);
temp = a;
a = b;
b = temp;
System.out.println(“After Number = “ + a + ” “ + b);
}
}
output
:- Enter Two Number = 10
20
before Number = 10 20
After Number = 20 10
Q.6 write a program to swap -2 ?
import java.util.Scanner;
class Swap
{
public static void main(String[] args) {
int a, b;
System.out.print("Enter
Two Number = ");
Scanner r = new Scanner(System.in);
a = r.nextInt();
b = r.nextInt();
System.out.println("before
Number = " + a + " " + b);
a = a + b;
b = a - b;
a = a - b;
System.out.println("After
Number = " + a + " " + b);
}
}
Output:-
Enter Two Number = 20
30
before Number = 20 30
After Number = 30 20
Q.7 W.A.P. to add two number ?
import java.util.Scanner;
class A {
public static void main(String[] args) {
int a, b, c;
Scanner s = new Scanner(System.in);
System.out.println(“Enter two number:”);
a = s.nextInt();
b = s.nextInt();
c = a + b;
System.out.print(“Sum of two number “ + c);
}
}
Output:-
Enter two number:
23
43
Sum of two number 66
Q.8 W.A.P. to calculate total marks and Average present marks of five subjects?
/*Find 5 subject present Value*/
import java.util.Scanner;
class present {
public static void main(String[] args) {
int a, b, c, d, e;
System.out.print(“Enter five subject Mask = “);
Scanner obj = new Scanner(System.in);
a = obj.nextInt();
b = obj.nextInt();
c = obj.nextInt();
d = obj.nextInt();
e = obj.nextInt();
float sum = a + b + c + d + e;
System.out.println(“Total Mask” + sum);
float average = sum / 5;
System.out.println(“Average / Present = ” + average);
}
}
Output:-
Enter five subject Mask = 78
98
89
90
87
Total Mask442.0
Average / Present = 88.4
Q.9 Write a Program to print ASCII value
/*Amarican standerds code for Information Intrachange */
/* Print ASCII. value of charactor */
// input A—–>65
import java.util.Scanner;
class ASCII
{
public static void main(String[] args)
{
char ch;
System.out.print(“Enter any character “);
Scanner r = new Scanner(System.in);
ch = r.next().charAt(0);
int a = ch;
System.out.print(“ASCII value of “ + ch + ” is “ + a);
}
}
Output:-
Enter any character A
ASCII value of A is 65
Enter any character B
ASCII value of B is 66
Q. what is Operater ? Its Types ?
Operator:- operator is a symbol that is used , to perform requirement.
- Arithmetic operator ( + , – , * , / ,% )
- Relational operator ( > , < , > = , < = , == , != )
- Logical operator ( && , II , ! )
- Assignment operator ( = , + = , -= , * = , /= )
- Ternary operator (? : )
- Bitwise operator ( & , I , ^ , ~ , << , >> )
Q. what is Arithmetic Operator ? with Program
Defination =Arithmetic operators are symbols used in programming and mathematics to perform basic arithmetic operations on numerical values. These operations include addition, subtraction, multiplication, division, and sometimes exponentiation, modulus (remainder of division), and integer division. Here’s a brief overview of each arithmetic operator:
Addition (+): Adds two operands.
- Example:
a + b
- Example:
Subtraction (-): Subtracts the second operand from the first.
- Example:
a - b
- Example:
Multiplication (*): Multiplies two operands.
- Example:
a * b
- Example:
Division (/): Divides the first operand by the second.
- Example:
a / b
- Example:
Exponentiation () (depending on the programming language)**: Raises the first operand to the power of the second.
- Example:
a ** b
- Example:
Modulus (%): Returns the remainder of the division of the first operand by the second.
- Example:
a % b
- Example:
Integer Division (//): Returns the integer part of the division of the first operand by the second.
- Example:
a // b
- Example:
Q. write a program to Arithmetic Operators ?
import java.util.Scanner;
class dk {
public static void main(String[] args) {
int a , b ;
System.out.println(“Enter two value:-“);
Scanner obj = new Scanner(System.in);
a = obj.nextInt();
b = obj.nextInt();
System.out.println(“sum= “ + (a + b));
System.out.println(“multiplication= “ + (a * b));
System.out.println(“subtraction= “ + (a – b));
System.out.println(“remender= “ + (a % b));
System.out.println(“division= “ + (a / b));
}
}
Enter two value:-
20
10
sum= 30
multiplication= 200
subtraction= 10
remender= 0
division= 2
Q. what is Relational Operators ? with Program.
Relational Operators relational operators are used to establish relationships or comparisons between two values.
Equal to (
==
): Checks if the values of two operands are equal.- Example:
a == b
- Example:
Not equal to (
!=
): Checks if the values of two operands are not equal.- Example:
a != b
- Example:
Greater than (
>
): Checks if the value of the left operand is greater than the value of the right operand.- Example:
a > b
- Example:
Greater than or equal to (
>=
): Checks if the value of the left operand is greater than or equal to the value of the right operand.- Example:
a >= b
- Example:
Less than (
<
): Checks if the value of the left operand is less than the value of the right operand.- Example:
a < b
- Example:
Less than or equal to (
<=
): Checks if the value of the left operand is less than or equal to the value of the right operand.- Example:
a <= b
- Example:
Equal to (
==
): Checks if the values of two operands are equal.- Example:
a == b
- Example:
Not equal to (
!=
): Checks if the values of two operands are not equal.- Example:
a != b
- Example:
Greater than (
>
): Checks if the value of the left operand is greater than the value of the right operand.- Example:
a > b
- Example:
Greater than or equal to (
>=
): Checks if the value of the left operand is greater than or equal to the value of the right operand.- Example:
a >= b
- Example:
Less than (
<
): Checks if the value of the left operand is less than the value of the right operand.- Example:
a < b
- Example:
Less than or equal to (
<=
): Checks if the value of the left operand is less than or equal to the value of the right operand.- Example:
a <= b
- Example:
Relational operator program 1
public class RelationalOperatorsExample {
public static void main(String[] args) {
int x = 10;
int y = 5;
// Relational operators
System.out.println(“x == y: ” + (x == y)); // false
System.out.println(“x != y: ” + (x != y)); // true
System.out.println(“x > y: ” + (x > y)); // true
System.out.println(“x >= y: ” + (x >= y)); // true
System.out.println(“x < y: ” + (x < y)); // false
System.out.println(“x <= y: ” + (x <= y)); // false
}
}
Relational operator user input program 2
import java.util.Scanner;
class dilip
{
public static void main(String[] args)
{
int a, b;
System.out.println(“Enter two value”);
Scanner obj = new Scanner(System.in);
a = obj.nextInt(); // greater
b = obj.nextInt(); // Lessar
System.out.println(“true/false= ” + (a < b));
System.out.println(“true/false= ” + (a > b));
System.out.println(“true/false= ” + (a <= b));
System.out.println(“true/false= ” + (a > =b));
System.out.println(“true/false= ” + (a == b));
System.out.println(“true/false= ” + (a != b));
}
}
Enter two value
20
10
true/false= true
true/false= false
true/false= true
true/false= false
true/false= true
Q. what is Logical Operators ? Full Explain ?
Logical Operators= Logical operators are used to perform operations on boolean expressions. These operators allow you to combine multiple boolean expressions or manipulate the boolean values themselves
Logical Operator Program 1
class LogicalOperator {
public static void main(String[] args) {
int a = 10, b = 20;
System.out.println(a == b && a != b);
System.out.println(a == b || a != b);
System.out.println(!(a > b));
}
}
output:-
false
true
true
Logical Operator Program 2
class logical {
public static void main(String[] args) {
System.out.println(“Logical And:- “);
System.out.println((10 > 5) && (2 > 1)); // true
System.out.println((10 > 5) && (2 < 1)); // false
System.out.println((10 < 5) && (2 < 1)); // false
System.out.println(“Logical OR:- “);
System.out.println((10 > 5) || (2 < 1)); // true
System.out.println((10 > 5) || (2 < 1)); // true
System.out.println((10 > 5) || (2 < 1)); // false
System.out.println(“Logical NOT:- “);
System.out.println(!(10 > 5)); // false
System.out.println(!(10 < 5)); // true
}
}
Output:-
Logical And:-
true
false
false
Logical OR:-
true
true
true
Logical NOT:-
false
true
Q. what is Assignment Operators ? Full Explain.
the assignment operator (=
) is used to assign a value to a variable.
Assignment Operator Program
class Assignment {
public static void main(String[] args) {
int a = 10; // simple
System.out.println(a);
a += 10; // compound
System.out.println(a);
a -= 10; // compound
System.out.println(a);
}
}
output:-
10
20
10
Q what is Ternary Operator ? Full Explain.
Ternary Operator= the ternary operator (also known as the conditional operator) ? :
is a shorthand notation for expressing conditional expressions. It provides a compact way to evaluate a condition and choose one of two possible values based on whether the condition evaluates to true or false.
Ternary Operator Program 1
class Ternary {
public static void main(String[] args) {
int a = 10, b = 20, max;
max = (a > b) ? a : b;
System.out.print(max);
}
}
Output:-20
Ternary Operator Program 2
public class B
{
public static void main(String[] args)
{
int a = 10, b = 20, c = 50;
int r = (a > b) ? (a > c ? a : c) : (b > c ? b : c);
System.out.println(r);
}
}
Output:-50
Ternary Operator using String
class TernaryStringExample {
public static void main(String[] args) {
int age = 20;
String status = (age >= 18) ? “Adult” : “Minor”;
System.out.println(“Person is a ” + status); // prints Person is a Adult
}
}
Q. what is Bitwise Operator ? full explain.
Bitwise = Defination bitwise operators are used to perform operations on individual bits of integer operands.
Bitwise Operator Program
Ans=
Step1àbinary concept ( 0 & 1 )
——32——-16——-8——-4———2———–1
10 ka binary 1 0 1 0 =1010
8 ka binary 1 0 0 0 =1000
17 ka binary 1 0 0 0 1 =10001
37 ka binary 1 0 0 1 0 1 =100101
5 ka binary 0 1 0 1 =0101
7 ka binary 0 1 1 1 =0111
2 ka binary 0 0 1 0 = 0010
6 ka binary 0 1 1 0 =0110
Step2à Table ( int a=5 ( 0101 ) , b=7 ( 0111 ) )
a | b | AND(&) | OR( | ) | Explor( ^ ) | Compliment(~) |
0 | 0 | 0 | 0 | 0 | 0 |
1 | 1 | 1 | 1 | 0 | 1 |
0 | 1 | 0 | 1 | 1 | 1 |
1 | 1 | 1 | 1 | 0 | 0 |
Total | 5 | 7 | 2 | 6 |
Compliment ~a
0101
+ 1
——-
0110
Bitwise Progra
class Bitwise {
public static void main(String[] args) {
int a = 5, b = 7;
System.out.println(“AND = “ + (a & b));
System.out.println(“OR = “ + (a | b));
System.out.println(“XOR = “ + (a ^ b));
System.out.println(“complement = “ + (~a));
}
}
Output:-
AND = 5
OR = 7
XOR = 2
complement = -6
Q. what is Conditional statement ? Full Explain
Defination= Use if to specify a block of code to be executed, if a specified condition is true. Use else to specify a block of code to be executed, if the same condition is false. Use else if to specify a new condition to test, if the first condition is false.
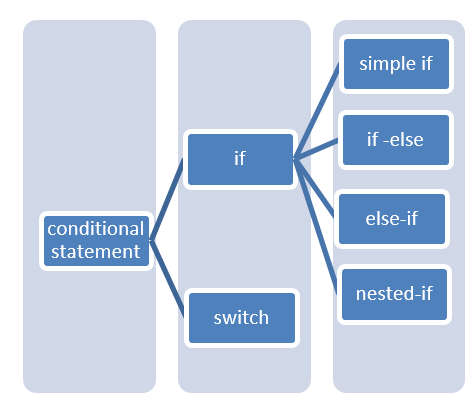
Q. what is Loop ? Full Explain.
Defination = loop is nothing but iterative statement which allow a block of code to be executed
repeatedly.
Types of Loops =
- For Loop
- While Loop
- do-while Loop
- Nested Loop
Q. what is Jump statement ? Full Explain.
Defination= Jump statements are used to transfer the control of the program to the specific statements. In other words, jump statements transfer the execution control to the other part of the program. There are two types of jump statements in Java, i.e., break and continue.
Types of Jump statements =
- continue
- break
Q. what is if statement ?
1. Simple if statement:- it is used when we want to test a single condition.
if statement में जब condition true होती तब code execute होता है और condition false होती है तो code execute नहीं होता |
Syntax:- if condition
{
//statement;
}
if flowchart
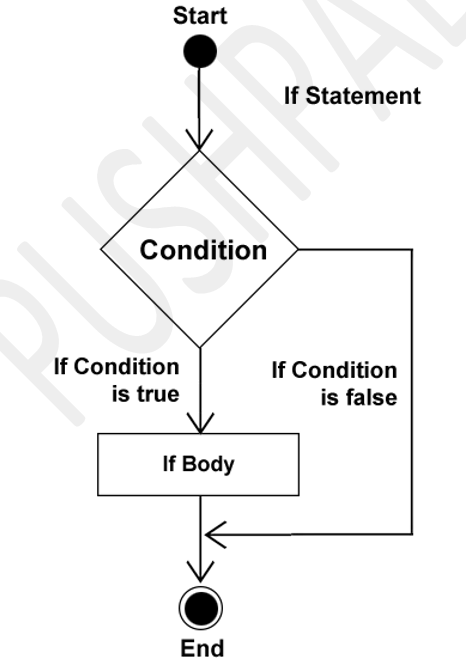
Q.1 write a program to find age vote person ?
import java.util.Scanner;
class simple {
public static void main(String[] args)
{
int age;
System.out.println(“Enter your age = “);
Scanner obj = new Scanner(System.in);
age = obj.nextInt();
if (age > 18)
{
System.out.println(“Elegible for vote”);
}
}
}
Output:-
Enter your age =
99
Elegible for vote
Q.2 write a program to find Number of greatest .
import java.util.Scanner;
class A
{
public static void main(String[] agrs)
{
Scanner r = new Scanner(System.in);
int n;
System.out.println(“Enter No:”);
n = r.nextInt();
if (n > 10)
{
System.out.println(“n is less than 10”);
}
}
}
Output:-
Enter No: 55
n is less than 10
3. write a program to create password using if statement ?
import java.util.Scanner;
class C
{
public static void main(String[] agrs)
{
Scanner r = new Scanner(System.in);
int pwd;
System.out.println(“Enter Password:-“);
pwd = r.nextInt();
if (pwd == 5810)
{
System.out.println(“Name:- Dilip Pushpad”);
System.out.println(“age 21”);
System.out.println(“contract NO.3545462625”);
System.out.println(“address – Indorea”);
}
}
}
Output:-
Enter Password:-
5810
Name:- Dilip Pushpad
age 21
contract NO.3545462625
address – Indore
Q. what is if else statement ?
Ans = It is used when we want to execute two statements for a single condition.
Note:- One of the two statements will be executed.
Syntax:-
if(condition)
{
//statement1;
}
else
{
//statement2;
}
else if flowchart
Q.1 Write a program to find positive and nagetive value using by if else statement ?
/* if else statement…! */
import java.util.Scanner;
class ifElse {
public static void main(String[] args) {
int n;
System.out.print(“Enter value = “);
Scanner obj = new Scanner(System.in);
n = obj.nextInt();
if (n >= 0) {
System.out.println(“Positive value”);
} else {
System.out.println(“Negitive value”);
}
}
}
Output:-
Enter value = 5
Positive value
Enter value = -10
Negitive value
Q.2 Write a program to create a mobile password using by if else ?
import java.util.Scanner;
class C {
public static void main(String[] agrs) {
Scanner r = new Scanner(System.in);
int pwd;
System.out.println(“Enter Password:-“);
pwd = r.nextInt();
if (pwd == 5810) {
System.out.println(“Name:- Dilip Pushpad”);
System.out.println(“age 21”);
System.out.println(“contract NO.3545462625”);
System.out.println(“address – Indore”);
} else {
System.out.println(“sorry! wrong password”);
}
}
}
Ouput:-
Enter Password:-
465
sorry! wrong password
Q.3 Write a program to odd even program in java ?
/* odd Even Program */
import java.util.Scanner;
class oddEven {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number: “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
if (n % 2 == 0) {
System.out.print(“Even Number”);
} else {
System.out.print(“Odd Number”);
}
}
}
Output:-
Enter any Number: 3
Odd Number
Enter any Number: 10
Even Number
Q.4 Write a program to Find a Maximum Number.
/* Maximum Number Print */
import java.util.Scanner;
class Max {
public static void main(String[] args) {
int a, b;
System.out.print(“Enter two value”);
Scanner r = new Scanner(System.in);
a = r.nextInt();
b = r.nextInt();
if (a > b)
{
System.out.print(“a=” + a);
}
else
{
System.out.print(“b=” + b);
}
}
}
Output:-
Enter two value10
20
b=20
Q.5 Write a program to check wovel and consonaut number ?
//ovel –> a,e,i,o,u.
//consonaut–>b,c,d,f.etc.
import java.util.Scanner;
class check {
public static void main(String[] args) {
char ch;
System.out.print(“Enter any Charactor: “);
Scanner r = new Scanner(System.in);
ch = r.next().charAt(0);
if (ch == ‘a’ || ch == ‘e’ || ch == ‘i’ || ch == ‘o’ || ch == ‘u’) {
System.out.print(“Vowel”);
} else {
System.out.print(“consonaut”);
}
}
}
Output:-
Enter any Charactor: a
Vowel
Enter any Charactor: b
Consonaut
Q.6 Write a program to vote system ?
/* vote System Program */
import java.util.Scanner;
class vote {
public static void main(String[] args) {
int age;
System.out.print(“Enter any age = “);
Scanner obj = new Scanner(System.in);
age = obj.nextInt();
if (age > 18) {
System.out.println(“eilagible for vote”);
} else {
System.out.print(“Not eilagible for Vote”);
}
}
}
Output:-
Enter any age = 17
Not eilagible for Vote
Enter any age = 20
eilagible for vote
Q.7 Write a program to convert character uppercase to
lowercase and vice - versa.
/* Convert character Lower to Upper & vice – versa */
// input a to z —–> Uppercase
// input A to Z —–> Lowercase
import java.util.Scanner;
class Convert {
public static void main(String[] args) {
char ch, ch2;
System.out.print(“Enter any character “);
Scanner r = new Scanner(System.in);
ch = r.next().charAt(0);
if (ch >= ‘A’ && ch <= ‘Z’) {
ch2 = Character.toLowerCase(ch);
System.out.print(“Lowercase “ + ch2);
} else {
ch2 = Character.toUpperCase(ch);
System.out.print(“Uppercase “ + ch2);
}
}
}
Output:-
Enter any character D
Lowercase d
Q.8 Write a program to find uppercase and lowercase.
/* Convert character Lower to Upper & vice – versa */
// input a to z —–> Uppercase
// input A to Z —–> Lowercase
import java.util.Scanner;
class Convert
{
public static void main(String[] args) {
char ch, ch2;
System.out.print(“Enter any character “);
Scanner r = new Scanner(System.in);
ch = r.next().charAt(0);
if (ch >= ‘A’ && ch <= ‘Z’) {
ch2 = Character.toUpperCase(ch);
System.out.print(“Uppercase “ + ch2);
} else {
ch2 = Character.toLowerCase(ch);
System.out.print(“Lowercase “ + ch2);
}
}
}
Output:-
Enter any character D
Uppercase D
Q.9 Write a program to print leap year ?
/* Leap Year Program */
// 1. century (100%=0 and 400%=0) 2000 2400 1700 1800 1900
// 2. yearly ( 100%!=0 and 4%=0) 2020 2024 2021 2022 2023
import java.util.Scanner;
class Year {
public static void main(String[] args) {
int y;
System.out.print(“Enter any Year”);
Scanner r = new Scanner(System.in);
y = r.nextInt();
if (y % 100 == 0 && y % 400 == 0) {
System.out.print(“Leap Year”);
} else {
System.out.print(“Not Leap Year”);
}
}
}
Output:-
Enter any Year2020
Leap Year
Enter any Year2022
Not Leap Year
Q.10 Write a program to check Divisibility of 5 ?
//input 10 –> Divisible by 5
//input 12 –> Not Divisible by 5
import java.util.Scanner;
class Divisible {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
if (n % 5 == 0) {
System.out.print(“Divisible by 5”);
} else {
System.out.print(“Not Divisible by 5”);
}
}
}
Output:-
Enter any Number 20
Not Divisible by 5
Q. what is else if statement ( else if lader ) ? Full explain.
Ans= it is used when we have only one if black , multiple else-if blocks.
Syntax:-
if (condition)
{
//statement 1;
}
{
Statement 2;
}
else
{
Statement 3;
}
Q. Flow char in else if statement ?
Q.1 Write a program to else if statement ?
/* ElseIfStatement.java */
class ElseIfStatement
{
public static void main(String args[])
{
int i = 5;
int j = 6;
if (i == j) {
System.out.println(“i is equal to j”);
} else if (i < j) {
System.out.println(“i is less than j”);
} else {
System.out.println(“i is greater than j”);
}
}
}
Output:-
i is less than j
Q.2 Write a Program to find student Division ?
import java.util.Scanner;
class condition_else_if {
public static void main(String[] agrs) {
int marks;
System.out.println(“Enter marks:-“);
Scanner r = new Scanner(System.in);
marks = r.nextInt();
if (marks >= 60 && marks < 100) {
System.out.println(“First Division”);
} else if (marks > 45 && marks < 60) {
System.out.println(“Second Division”);
} else if (marks >= 33 && marks < 45) {
System.out.println(“third division”);
} else {
System.out.println(“Failed”);
}
}
}
Output:-
Enter marks:-
50
Second Division
Q.3 Write a program to calculate tax of a salary ?
/* Tax calculation Program */
//input sal<=10,000 —-> No tax
//input sal>10,000 b/w sal<=100,000——> 10% tax
//input sal>100,000——–>20% tax
import java.util.Scanner;
class Tax {
public static void main(String[] args) {
int sal;
double tax;
System.out.print(“Enter salary “);
Scanner obj = new Scanner(System.in);
sal = obj.nextInt();
if (sal <= 10000) {
System.out.print(“No Tax”);
} else if (sal > 10000 && sal <= 100000) {
tax = sal * 0.10;
System.out.print(“tax = “ + tax);
} else {
tax = sal * 0.20;
System.out.print(“tax = “ + tax);
}
}
}
Output=
Enter salary 10000
No Tax
Enter salary 230000
tax = 46000.0
Q. What is nested if - else ? Full Explain.
Ans= whenever , we define if – else block inside anothors if –else block called nested if-else.
Syntax:-
if(condition 1)
{
if(condition 2)
{
//code;
}
else
{
//code;
}
}
else
{
if(condition 3)
{
//code;
}
else
{
//code;
}
}
Q.1 Write a program to nested if else ?
class Nested {
public static void main(String[] args)
{
int a = 10, b = 20, c = 30;
if (a > b)
{
if (a > b)
{
System.out.println(a);
}
else
{
System.out.println(c);
}
}
else
{
if (b > c)
{
System.out.println(b);
}
else
{
System.out.println(c);
}
}
}
}
Output:- 30
Q.2 write a program to find Greated of three number
/* nested if statement */
import java.util.Scanner;
class nestedif {
public static void main(String[] args) {
int a, b, c;
System.out.print(“rahul age=”);
Scanner obj = new Scanner(System.in);
a = obj.nextInt();
System.out.print(“Mohit age=”);
b = obj.nextInt();
System.out.print(“Ram age=”);
c = obj.nextInt();
if (a > b) {
if (a > b) {
System.out.println(“Rahul big age=” + a);
} else {
System.out.println(“Mohit big age=” + b);
}
} else {
if (b > c) {
System.out.println(“Mohit big age=” + b);
} else {
System.out.println(“Ram big age=” + c);
}
}
}
}
Output:-
rahul age=20
Mohit age=50
Ram age=30
Mohit big age=50
Q.3 Write a program to make calculator logic ?
/* Make calculator */
import java.util.Scanner;
class calculator {
public static void main(String[] args) {
int a, b, ch, cal;
System.out.print(“Enter Two value = “);
Scanner r = new Scanner(System.in);
a = r.nextInt();
b = r.nextInt();
System.out.print(“Select Operation “);
ch = r.nextInt();
if (ch == 1) {
cal = a + b;
System.out.print(“sum = “ + cal);
} else if (ch == 2) {
cal = a – b;
System.out.print(“sub = “ + cal);
} else if (ch == 3) {
cal = a * b;
System.out.print(“multi = “ + cal);
} else if (ch == 4) {
cal = a / b;
System.out.print(“div = “ + cal);
} else if (ch == 5) {
cal = a % b;
System.out.print(“remender = “ + cal);
} else {
System.out.print(“Invalid choice”);
}
}
}
Output : –
Enter Two value = 50
30
Select Operation 2
sub = 20
Q.4 Write a proram to input month number and print number of days ?
/* Enter.month.number & print number of days in a month */
import java.util.Scanner;
class month {
public static void main(String[] args) {
int n;
System.out.print(“Enter Number: “);
Scanner s = new Scanner(System.in);
n = s.nextInt();
if (n == 1) {
System.out.print(“Jan = 31 Days”);
} else if (n == 2) {
System.out.print(“Feb = 28 Days”);
} else if (n == 3) {
System.out.print(“Merch = 31 Days”);
} else if (n == 4) {
System.out.print(“April = 30 Days”);
} else if (n == 5) {
System.out.print(“May = 31 Days”);
} else if (n == 6) {
System.out.print(“June = 30 Days”);
} else if (n == 7) {
System.out.print(“July = 31 Days”);
} else if (n == 8) {
System.out.print(“Aug = 31 Days”);
} else if (n == 9) {
System.out.print(“Sept = 30 Days”);
} else if (n == 10) {
System.out.print(“oct = 31 Days”);
} else if (n == 11) {
System.out.print(“Nov = 30 Days”);
} else if (n == 12) {
System.out.print(“Dec = 31 Days”);
} else {
System.out.print(“Invalid choice”);
}
}
}
Output:-
Enter Number: 4
April = 30 Days
Q.5 Write a program to check number is positive of Nagative ?
/* Check number is positive of Nagative */
//input n>0 +ve Number
// input n=0 Neither +ve Nor -ve
import java.util.Scanner;
class ckeck {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
if (n > 0) {
System.out.print(“+ve Number”);
} else if (n < 0) {
System.out.print(“-ve Number”);
} else {
System.out.print(“Niether +ve nor -ve Number”);
}
}
}
Output:-
Enter any Number 7
+ve Number
Enter any Number -65
-ve Number
Q. What is Switch statement ? Full Explain.
Defination :- Switch
is a multiple choice decision making selection statement , it used when we want
to select only one case out of multiple case.
Syntax:-
switch (key)
{
case 1: statement 1;
break;
case 2: statement 2;
break;
case 3: statement 3;
break;
default:
break;
}
Flowchar in Switch
Q.1 Write a program to find Vowel and consonant value ?
import java.util.Scanner;
class B {
public static void main(String[] args) {
char n;
System.out.println(“Enter charactor”);
Scanner r = new Scanner(System.in);
n = r.next().charAt(0);
switch (n)
{
case ‘a’:
case ‘e’:
case ‘i’:
case ‘o’:
case ‘u’:
System.out.println(“vowel”);
break;
default: {
System.out.println(“consonant”);
}
}
}
}
Output:-
Enter charactor
i
vowel
Enter charactor
q
consonant
Q.2 Write a program to make calculator ?
import java.util.Scanner;
class switch_program {
public static void main(String[]args)
{
int a=10,b=20,ch;
System.out.println(“Enter user choice..!”);
Scanner r=new Scanner(System.in);
ch=r.nextInt();
switch (ch)
{
case 1: System.out.println(“sum”+(a+b));
break;
case 2: System.out.println(“sub”+(a–b));
break;
case 3: System.out.println(“multi”+(a*b));
break;
case 4: System.out.println(“div”+(a/b));
break;
default: System.out.println(“Invalid choice…”);
break;
}
}
}
Output:- Enter user choice..!
1
Enter user choice..!
5
nvalid choice…
Q. What is Loop ? Full Explain.
Loop :- loop is nothing but iterative statement which allow a block of code to be executed
repeatedly,
एक ही statement को बार–बार repeat करना ‘Looping’ होता है |
Tyeps of loops:-
· while loop
· do while loop
· for loop
· nested loop
Q. What is While ? Full Explain.
while loop:- while loop is pre – test loop. It used when we don’t known the number of
iterations in advance.
It is also known of Entry control loop.
while Loop जब तक condition true होती है तब तक loop repeat होता रहता है | अगर condition false होता है, तब Loop exit हो जाता है |
while loop Syntax :-
//variable_initialization;
while( condition )
{
//statement(s);
increment/decrement;
}
while loop Flowchart
Q.1 Write a program to while loop ?
//WhileLoop.java
class WhileLoop{
public static void main(String args[]) {
int i = 0;
while(i < 10){
System.out.println("Value of i is " + i);
i++;
}
}
}
Output:-
Value of i is 0
Value of i is 1
Value of i is 2
Value of i is 3
Value of i is 4
Value of i is 5
Value of i is 6
Value of i is 7
Value of i is 8
Value of i is 9
Q.2 Write a program to prime name more than times ?
import java.util.Scanner;
class First {
public static void main(String[] args) {
int n;
System.out.println(“Enter value for condition”);
Scanner r = new Scanner(System.in);
n = r.nextInt();
while (n >= 0) {
System.out.println(“Pushpad computer”);
}
}
}
Output:-
Pushpad computer
Pushpad computer
Pushpad computer
Q.3 Write a program to find sum of Degits ?
/* Reverse Number Program */
//input number 123 —-> 321
import java.util.scanner;
class reverse {
public static void main(String[] args) {
int n, r;
System.out.print(“Enter any Number “);
Scanner ref = new Scanner(System.in);
n = ref.nextInt();
while (n > 0) {
r = n % 10;
System.out.print(“Reverse Order “ + r);
n = n / 10;
}
}
}
Output:-
Enter any Number 158
sum of Digits 14
Q.4 Write a program to Count Degits in a number ?
/* count of Number digits */
// input 435454 —-> 6
import java.util.Scanner;
class digits {
public static void main(String[] args) {
int n, count = 0;
System.out.print(“Enter any Digits = “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
while (n > 0) {
n = n / 10;
count++;
}
System.out.print(“Count of Digits = “ + count);
}
}
Output:-
Enter any Digits = 761026
Count of Digits = 6
Q What is do-while Loop ? Full Explain.
Do while Loop:- do while loop is a post-test loop, it is used when we want to execute loop body at least once even condition is false.
It is also known as exit control loop.
Syntax:-
Do
{
//statements;
}
While(condition);
flowchart do-while Loop :-
Q.1 Write a Program to do-while loop ?
class DoWhileLoop
{
public static void main(String args[])
{
int i = 0;
do
{
System.out.println("Value of i is " + i);
i++;
}
while(i < 10);
}
}
Output:-
Value of i is 0
Value of i is 1
Value of i is 2
Value of i is 3
Value of i is 4
Value of i is 5
Value of i is 6
Value of i is 7
Value of i is 8
Value of i is 9
Q.2 Write a Program to print value condition according ?
import java.util.Scanner;
class First {
public static void main(String[] args) {
int n = 1;
do {
System.out.println(n);
++n;
} while (n < 0);
}
}
Output:- 1
Q.3 Write a Program to find of sum positive nubmers ?
// Java program to find the sum of positive numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int sum = 0;
int number = 0;
// create an object of Scanner class
Scanner input = new Scanner(System.in);
// do…while loop continues
// until entered number is positive
do {
// add only positive numbers
sum += number;
System.out.println(“Enter a number”);
number = input.nextInt();
} while(number >= 0);
System.out.println(“Sum = ” + sum);
input.close();
}
}
output:-
Enter a number
25
Enter a number
25
Enter a number
-1
Sum = 50
Q. what is for loop ? Full explain.
for loop= unlike while loop , for loop performs all operation , in single line.
Syntax:- for (initialization, condition, incr/deer.)
{
//block of code;
}
Flowchart for loop
Q.1 Write a program to for loop ?
class For_loop {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
System.out.println(i);
}
}
}
Output:-
1
2
3
4
5
6
7
8
9
10
Q.2 Write a Program to print A to Z alphabets.
/* Alphabet Print Program */
// A B C D……………Z
class Alphabet {
public static void main(String[] args) {
for (char i = ‘A’; i <= ‘Z’; i++)
{
System.out.print(i + ” “);
}
}
}
Output:-
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Q.3 write a program to for loop ?
class ForLoop{
public static void main(String args[]){
for( int i=0; i < 10; i++ ){
System.out.println("Value of i is " + i);
}
}
}
output:-
Value of i is 0
Value of i is 1
Value of i is 2
Value of i is 3
Value of i is 4
Value of i is 5
Value of i is 6
Value of i is 7
Value of i is 8
Value of i is 9
Q.4 Write a Program to print nutural Number.
/* Natural Number Program */
import java.util.Scanner;
class Nutural {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number: “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
for (int i = 1; i <= n; i++) {
System.out.print(i + ” “);
}
}
}
Output:-
Enter any Number: 10
1 2 3 4 5 6 7 8 9 10
Q.5 Write a program to print odd number.
/* Odd Number Program */
import java.util.Scanner;
class Odd {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number: “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
for (int i = 1; i <= n; i = i + 2) {
System.out.print(i + ” “);
}
}
}
Output:-
Enter any Number: 20
1 3 5 7 9 11 13 15 17 19
Q.6 Write a program to print even number?
/* Even Number Program */
import java.util.Scanner;
class Even {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number: “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
for (int i = 2; i <= n; i = i + 2) {
System.out.print(i + ” “);
}
}
}
Output:-
Enter any Number: 20
2 4 6 8 10 12 14 16 18 20
Q.7 Write a program to Find factorial of a number ?
/* Find factorial of a Number */
// input 5–à120 (5*4*3*2*1)
import java.util.Scanner;
class factorial {
public static void main(String[] args) {
int n, fact = 1;
System.out.print(“Enter any Number = “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
for (int i = 1; i <= n; i++) {
fact = i * fact;
}
System.out.print(“factorial Number = “ + fact);
}
}
Output:-
Enter any Number = 5
factorial Number = 120
Q.8 Write a program to calculate power of number ?
/* Calculate Power of Number */
import java.util.Scanner;
class Power {
public static void main(String[] args) {
int n, p, result = 1;
System.out.print(“Enter Number “);
Scanner r = new Scanner(System.in);
n = r.nextInt(); //5
System.out.print(“Enter Power “);
p = r.nextInt(); //4
for (int i = 1; i <= p; i++) {
result = n * result; //5*5*5*5
}
System.out.print(“Power calculate=” + result);
}
}
Output:-
Enter Number 5
Enter Power 4
Power calculate=625
Q.9 Write a program to print multiplication table?
/* Print Multiplication Table */
import java.util.Scanner;
class Table {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number = “);
Scanner obj = new Scanner(System.in);
n = obj.nextInt();
for (int i = 1; i <= 10; i++) {
System.out.println(n + “*” + i + “=” + n * i);
}
}
}
Output:-
Enter any Number = 10
10*1=10
10*2=20
10*3=30
10*4=40
10*5=50
10*6=60
10*7=70
10*8=80
10*9=90
10*10=100
Q.10 write a program find factor of a number ?
//input 10 —-> 1 2 5 10
import java.util.Scanner;
class factor {
public static void main(String[] args) {
int n;
System.out.print(“Enter any Number:- “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
for (int i = 1; i <= n; i++) {
if (n % i == 0) {
System.out.print(i + ” “);
}
}
}
}
Output:-
Enter any Number:- 20
1 2 4 5 10 20
Q what is for each loop ?
for each loop mainly used to fetch the value from a collection like array.
Syntax:-
For(datatype var 1:var 2)
{
//code;
}
Q Flowchat for each loop
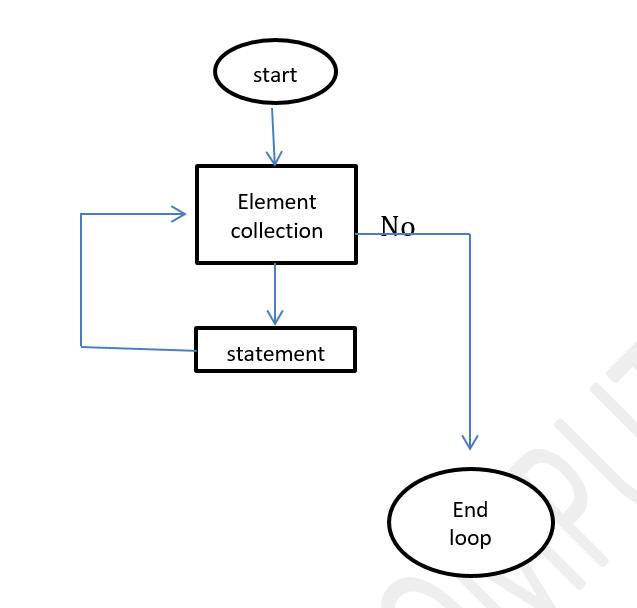
Q.1 write a program to foreach loop?
import java.util.Scanner;
class First {
public static void main(String[] args) {
int a[] = { 10, 20, 30, 40, 50 };
for (int b : a) {
System.out.println(b);
}
}
}
Output:-
10
20
30
40
50
Q. what is nested for loop?
Defination = A for loop which contain insid another for loop is called nested for loop.
Syntax:-
for(initialization;condition;incr/decre)
{
for(initialization;condition;incr/decre)
{
//code
}
}
1. Solid Square Star Pattern
/*Nested for loop solid square star pattern*/
class Nested {
public static void main(String[] args) {
int i, j;
for (i = 1; i <= 5; i++) {
for (j = 1; j <= 5; j++) {
System.out.print(“* “);
}
System.out.println();
}
}
}
Output:-
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
2. Half Pyramid Star Pattern
/*Star Pattern Program */
class A {
public static void main(String[] args) {
int i, j;
for (i = 1; i <= 5; i++) {
for (j = 1; j <= i; j++) {
System.out.print(” * “);
}
System.out.print(“\n”);
}
}
}
3. Inverted Half Pyramid Star Pattern 1
/*Star Pattern Program */
class A {
public static void main(String[] args) {
int i, j;
for (i = 1; i <= 5; i++) // raws
{
for (j = 5; j >= i; j–) // columns
{
System.out.print(” * “);
}
System.out.print(“\n”);
}
}
}
4. Inverted Half Pyramid Star Pattern 2
/*Star Pattern Program */
class A {
public static void main(String[] args) {
int i, j;
for (i = 5; i >= 1; i–) // raws
{
for (j = 1; j<=i; j++) // columns
{
System.out.print(” * “);
}
System.out.print(“\n”);
}
}
}
5. Inverted Half Pyramid Star Pattern - 3
/*Star Pattern Program */
class A {
public static void main(String[] args) {
int i, j;
for (i = 5; i >= 1; i–) // raws
{
for (j = 1; j<=i; j++) // columns
{
System.out.print(” * “);
}
System.out.print(“\n”);
}
}
}
6. inverted half Pyramid Star Pattern ( rotated by 180 degree )
/*Star Pattern Program */
class A {
public static void main(String[] args) {
int i, j, k;
for (i = 1; i <= 5; i++) // raws
{
for (j = i; j <= 4; j++) // space
{
System.out.print(” “);
}
for (k = 1; k <= i; k++) // columns
{
System.out.print(“*”);
}
System.out.print(“\n”);
}
}
}
7. Half Pyramid with Number Star Pattern - 1
/* Number Pattern (Logic) */
class A {
public static void main(String[] args) {
int i, j;
for (i = 1; i <= 5; i++) // raws
{
for (j = 1; j <= i; j++) // column
{
System.out.print(j+” “);
}
System.out.print(“\n”);
}
}
}
8. Half Pyramid with Number Star Pattern - 2
/* Number Pattern (Logic) */
class A {
public static void main(String[] args) {
int i, j;
for (i = 1; i <= 5; i++) // raws
{
for (j = 1; j <= i; j++) // column
{
System.out.print(i);
}
System.out.print(“\n”);
}
}
}
9. Inverted Half Pyramid with Number Star Pattern
/* Number Pattern (Logic) */
class A {
public static void main(String[] args) {
int i, j;
for (i = 5; i >= 1; i–) // raws
{
for (j = 1; j <= i; j++) // column
{
System.out.print(i);
}
System.out.print(“\n”);
}
}
}
10. Triangle 01 Star Pattern
/* Number Pattern (Logic) */
class A {
public static void main(String[] args) {
int i, j;
for (i = 1; i <= 5; i++) // raws
{
for (j = 1; j <= i; j++) // column
{
int sum=i+j;
if(sum%2==0)
{
System.out.print(“1”);//even
}
else{
System.out.print(“0”);//odd
}
}
System.out.print(“\n”);
}
}
}
11. Diamond star Pattern
/* Print Diamond Pattern */
class A {
public static void main(String[] args) {
int i, j, k;
for (i = 1; i <= 5; i++) // raws
{
for (j = 5; j > i; j–) // space(column)
{
System.out.print(” “);
}
for (k = 1; k <= (2 * i – 1); k++) {
System.out.print(“*”);
}
System.out.print(“\n”);
}
for (i = 4; i >= 1; i–) // raws
{
for (j = 5; j > i; j–) // space(column)
{
System.out.print(” “);
}
for (k = 1; k <= (2 * i – 1); k++) {
System.out.print(“*”);
}
System.out.print(“\n”);
}
}
}
12. Half-Diamond star pattern ?
/* Print Half-Diamond Pattern */
class A {
public static void main(String[] args) {
int i, j;
for (i = 1; i <= 5; i++) {
for (j = 1; j <= i; j++) {
System.out.print(“* “);
}
System.out.println();
}
for (i = 1; i <= 4; i++) {
for (j = 4; j >= i; j–) {
System.out.print(“* “);
}
System.out.println();
}
}
}
Output:-
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
13. Reverse Pyramid Star Pattern-1
class ReversePyramid
{
public static void main(String[] args)
{
int rows = 6; // Number of Rows we want to print
//Printing the pattern
for (int i = 1; i <= rows; i++)
{
for (int j = 1; j < i; j++)
{
System.out.print(” “);
}
for (int j = i; j <= rows; j++)
{
System.out.print(j+” “);
}
System.out.println();
}
}
}
14. Reverse pyramid Star Pattern-2
class ReversePattern
{
public static void main(String[] args)
{
int rows = 9; // Number of Rows we want to print
//Printing the pattern
for (int i = 1; i <= rows; i++)
{
for (int j = 1; j < i; j++)
{
System.out.print(” “);
}
for (int j = i; j <= rows; j++)
{
System.out.print(j+” “);
}
System.out.println();
}
//Printing the reverse pattern
for (int i = rows-1; i >= 1; i–)
{
for (int j = 1; j <= i; j++)
{
System.out.print(” “);
}
for (int j = i; j <= rows; j++)
{
System.out.print(j+” “);
}
System.out.println();
}
}
}
output:-
15. hellow Rectangle star pattern
//Print star hollow Rectangle pattern
class hellow_Rectangle
{
public static void main(String[]args)
{
for(int i=1;i<=4;i++)
{
for(int j=1;j<=5;j++)
{
if(i == 1 || j == 1 || i == 4 || j == 5)
{
System.out.print(“*”);
}
else{
System.out.print(” “);
}
}
System.out.println();
}
}
}
output:-
*****
* *
* *
*****
16. character triangle Star Pattern 1
/* Print character pattern */
class A {
public static void main(String[] args) {
char i, j;
for (i = ‘A’; i <= ‘E’; i++) {
for (j = ‘A’; j <= i; j++) {
System.out.print(j);
}
System.out.print(‘\n‘);
}
}
}
Output:-
A
AB
ABC
ABCD
ABCDE
17. character triangle Star Pattern 2
/* Print character pattern */
class A
{
public static void main(String[] args)
{
char i, j;
for (i = ‘A’; i <= ‘F’; i++)
{
for (j = ‘A’; j <= i; j++)
{
System.out.print(i);
}
System.out.print(‘\n‘);
}
}
}
Output:-
A
BB
CCC
DDDD
EEEEE
FFFFFF
18. Heart Star Pattern
class HelloWorld {
public static void main(String[] args)
{
for(int i=0;i<=5;i++)
{
for(int j=0;j<=6;j++)
{
if((i==0&&j%3!=0) || (i==1&&j%3==0) || i-j==2 || i+j==8)
{
System.out.print(“*”+” “);
}
else
{
System.out.print(” “+” “);
}
}
System.out.println();
}
}
}
output:-
* * * *
* * *
* *
* *
* *
*

Q. write a program java
Q. W.A.P. java Program to check number
is Armstrong or not ?
ADVANCE String Topic
String in Java
Defination = Java string are object that allows us to store sequence of character is called string.
Example:- char[ ] c={‘P’ , ‘U’ , ‘S’ , ‘H’ , ‘P’ , ‘A’ , ‘D’ };
String s = new String(c); //String
String a=”Dilip Pushpad”;
- which may contain alpha numeric value enclosed in double quotes (“ Dilip3 ”).
Note:-
- String are immutable in java.
- String is a class.
- String is non-primitive datatype.
- It contains methods that can perform certain operations on string. – ( concat() , equals() , length() etc..)
1. String
2. StringBuffer
3. StrigBuilder
There are two ways to create string object:-
- String literal
- new keyword
String Literal = String literals are sequences of characters enclosed between double quote (“”) marks. These characters can be alphanumeric, special characters, blank spaces, etc. Examples: “John”, “2468”, “\n”, etc.
Program Literal 1
class AB {
public static void main(String[] args) {
String a = “Pushpad”; // literal
System.out.println(a);
}
}
Output:- Pushpad
Program Literal 2
class B {
public static void main(String[] args) {
String a = “dilip”; // literal
System.out.println(a);
String b = “dilip”; // literal
System.out.println(b);
}
}
Output:– dilip
dilip
Program immutable concepts 3
class B {
public static void main(String[] args) {
String a = “dilip”; // literal
System.out.println(a);
String b = “dilip”; // literal
System.out.println(b);
a.concat(“pushpad”); // immutable concept
System.out.println(a);
}
}
output:-
dilip
dilip
dilip
concat using in string:-
class B {
public static void main(String[] args) {
String a = “dilip”; // literal
System.out.println(a);
String b = “dilip”; // literal
System.out.println(b);
a = a.concat(“pushpad”); // immutable concept
System.out.println(a);
}
}
output:-
dilip
dilip
dilippushpad
Q.1 Write a Program new keyword in string
class B {
public static void main(String[] args) {
String a = new String(“ankit”);
System.out.println(a);
String b = new String(“ankit”);
System.out.println(b);
}
}
Q.2 Write a Program new keyword concat() ( immutable )
class B {
public static void main(String[] args) {
String a = new String(“ankit”);
System.out.println(a);
String b = new String(“ankit”);
System.out.println(b);
a.concat(“kumar”);
System.out.println(a);
}
}
Output:-
ankit
ankit
ankit
Q.3 Write a Program to new keyword using cancat() method ?
class B {
public static void main(String[] args) {
String a = new String(“ankit”);
System.out.println(a);
String b = new String(“ankit”);
System.out.println(b);
a = a.concat(“kumar”);
System.out.println(a);
}
}
Output:-
ankit
ankit
ankitkumar
Q.4 Write a Program to string Literal ?
class B {
public static void main(String[] args) {
String a = new String(“ankit”);
System.out.println(a);
String b = new String(“ankit”);
System.out.println(b);
a = “kumar”;
System.out.println(a);
}
}
Output:-
ankit
ankit
kumar
Q.5 Write a Program to find string length ?
class stringClass {
public static void main(String[] args) {
String str = “dilip pushpad”;
int l = str.length();
System.out.print(l);
}
}
Output:-
13
Q.6 Write a Program to change lower to upper case ?
class stringClass
{
public static void main(String[] args) {
String str = “Dilip pushpad”;
System.out.print(str.toUpperCase());
}
}
Output:-
DILIP PUSHPAD
Q.7 Write a Program to change upper to lower case ?
class stringClass {
public static void main(String[] args) {
String str = “DILIP PUSHPAD”;
System.out.print(str.toLowerCase());
}
}
Output:-
dilip pushpad
Q.8 Write a Program to compare to string in java?
class stringClass {
public static void main(String[] args) {
String str = “DILIP”;
String str2 = new String(“DILIP”);
if (str.equals(str2))
{
System.out.print(“Both are equals”);
}
else
{
System.out.print(“not equals”);
}
System.out.print(” “);
}
}
Output:- Both are equals
Q.9 Write a Program to compair to string in java?
class Bb
Q.10 Write a Program to compair to string in java?
class Bb
Q.11 Write a Program to equals method in String ?
class Bb
Q.12 Write a Program to equals method in String ?
class Bb
Q.13 Write a Program to equals method in String ?
Q.14 String Basic Methods Program ?
class Bb
SHYAM
ShyamRam
5
Dilip
true
false
h
1
false
Malakar
Q.1 Write a program java program to check number is
armstrong or not ?
/* Armstrong Number Program */
//input number 153 —> (1*1*1 + 5*5*5 + 3*3*3 = 153)
import java.util.Scanner;
class A {
public static void main(String[] args) {
int n, arm = 0, rem, c;
System.out.print(“Enter any Number “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
c = n;
while (n > 0) {
rem = n % 10;
arm = (rem * rem * rem) + arm;
n = n / 10;
}
if (c == arm) {
System.out.print(“Armstrong Number”);
} else {
System.out.print(“Not Armstrong Number”);
}
}
}
Output:-
Enter any Number 153
Armstrong Number
Q.2 write a program check number is prime or not.
/* Prime Number Program */
// input number 7 —> Prime number
import java.util.Scanner;
class A {
public static void main(String[] args) {
int n, count = 0;
System.out.print(“Enter any Number “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
for (int i = 1; i <= n; i++)
{
if (n % i == 0)
{
count++;
}
}
if (count == 2)
System.out.print(“Prime Number”);
else
System.out.print(“Not Prime Number”);
}
}
Output:-
Enter any Number 7
Prime Number
Enter any Number 10
Not Prime Number
Q.3 write a program to program to check weather a number is perfect or not.
/* Perfect Number Program */
// 6 —-> 1 + 2 + 3 + 4 + 5
// 6 —> 1 + 2 + 3 =6
import java.util.Scanner;
class A {
public static void main(String[] args) {
int n, sum = 0;
System.out.print(“Enter any Number “);
Scanner r = new Scanner(System.in);
n = r.nextInt();
for (int i = 1; i < n; i++) {
if (n % i == 0) {
sum = sum + i;
}
}
if (n == sum)
System.out.print(“Perfect Number “);
else
System.out.print(“Not Perfect Number “);
}
}
Output:-
Enter any Number 6
Perfect Number
Enter any Number 15
Not Perfect Number
Q.4 write a program to print between two number ?
/* Prime b/w two Number */
//input first number 10
//inpupt second number 20
// Prime number —> 11 13 17 19
import java.util.Scanner;
class A {
public static void main(String[] args) {
int n1, n2, i, j;
System.out.print(“Enter Two Number “);
Scanner r = new Scanner(System.in);
n1 = r.nextInt();
n2 = r.nextInt();
for (i = n1; i <= n2; i++) {
for (j = 2; j <= i; j++)
{
if (i % j == 0)
break;
}
if (i == j)
System.out.print(j + ” “);
}
}
}
Output:-
Enter Two Number 10
20
11 13 17 19
Q.5 write a program to reverse a number ?
/* Reverse Number Program */
//input number 123 —> 321
import java.util.Scanner;
class Reverse {
public static void main(String[] args) {
int n, r;
System.out.print(“Enter any Number “);
Scanner ref = new Scanner(System.in);
n = ref.nextInt();
while (n > 0) {
r = n % 10;
System.out.print(r);
n = n / 10;
}
}
}
Output:-
Enter any Number 123
321
2. StringBuffer class
String objects are immutable and stringbuffer objects are mutable.
Example = StringBuffer sb = new StringBuffer(“Dilip”);
Sb.append(“java”);
Output = Dilip java
StringBuffer methods
Q.1 Write a program to StringBuffer Constractor ?
public class StringBufferExample
{
public static void main(String[] args)
{
StringBuffer sb = new StringBuffer();
System.out.print(sb.capacity());
}
}
Q.2 Write a program to StringBuffer constractor ?
public class StringBufferExample
{
public static void main(String[] args)
{
StringBuffer sb = new StringBuffer(“Dilip_sir”);
System.out.print(sb.capacity()); // 16 + string length 9 = 25
}
}
Q.3 Write a program to StringBuffer constractor ?
public class StringBufferExample
{
public static void main(String[] args)
{
StringBuffer sb = new StringBuffer(10000);
System.out.print(sb.capacity());
}
}
Q.4 Write a program to StringBuffer capacity method ?
public class StringBufferExample
{
public static void main(String[] args)
{
StringBuffer sb = new StringBuffer();
System.out.println(sb.capacity()); //16
sb.append(“Dilip”);
System.out.println(sb.capacity()); //16
sb.append(“Dilip Pushpad”);
System.out.println(sb.capacity()); // (old capacity 16 * 2)+2 = 34
sb.append(“Dilip Pushpad computer classes indore”);
System.out.println(sb.capacity()); // (old capacity 34 * 2)+2 = 70
}
}
output:-
16
16
34
70
Q.5 Write a program to StringBuffer methods?
public class StringBufferExample
{
public static void main(String[] args)
{
StringBuffer sb = new StringBuffer(“Dilip pushpad”);
// append method
System.out.println(sb.append(” Indore”));
StringBuffer sb2 = new StringBuffer(“pushpad”);
//charAt method
System.out.println(sb2.charAt(4));
StringBuffer sb3 = new StringBuffer(“Pushpad Computer”);
//delete method
System.out.println(sb3.delete(3,5));
StringBuffer sb4 = new StringBuffer(“Dilip Sir”);
//deleteCharAt method
System.out.println(sb4.deleteCharAt(2));
StringBuffer sb5 = new StringBuffer(“Dilip Sir”);
StringBuffer sb6 = new StringBuffer(“Dilip Sir”);
//equal method
System.out.println(sb5.equals(sb6));
StringBuffer sb7 = new StringBuffer(“Dilip”);
//equal method
StringBuffer sb8 = sb7.append(“sir”);
System.out.println(sb7.equals(sb8));
StringBuffer sb9 = new StringBuffer(“dilipp Pushpad”);
//replace method
System.out.println(sb9.replace(5,7,”sir”));
StringBuffer sb10 = new StringBuffer(“dilip Pushpad”);
//indexOf method
System.out.println(sb10 .indexOf(“P”));
StringBuffer sb11 = new StringBuffer(“Pushpad”);
//lastindexOf method
System.out.println(sb11.lastIndexOf(“a”));
StringBuffer sb12 = new StringBuffer(“Pushpad”);
//insert method
System.out.println(sb12.insert(7,”Computer”));
StringBuffer sb13 = new StringBuffer(“Pushpad Sir”);
//reverse method
System.out.println(sb13.reverse());
StringBuffer sb14 = new StringBuffer(“Dilip Pushpad”);
//subSequence method
System.out.println(sb14.subSequence(3,7));
StringBuffer sb15 = new StringBuffer(“Pushpad”);
//substring method
System.out.println(sb15.substring(3));
StringBuffer sb16 = new StringBuffer(“Pushpad”);
System.out.println(sb16.capacity());
//Ensure capacity method
sb16.ensureCapacity(1000);
System.out.println(sb16.capacity());
StringBuffer sb17 = new StringBuffer(“Pushpad”);
//setCharAt method
sb17.setCharAt(4,’d’);
System.out.println(sb17);
StringBuffer sb18 = new StringBuffer(“Pushpad”);
//length method
sb18.setLength(5);
System.out.println(sb18);
StringBuffer sb19 = new StringBuffer(“dilip”);
sb19.ensureCapacity(100);
System.out.println(sb19.capacity());
//substring method
sb19.trimToSize();//remove extra memory
System.out.println(sb19.capacity());
}
}
output:-
Q. what is StringBuilder ?
StringBuilder is a mutable and non synchronized.
StringBuilder methods
Q.1 Write a program to StringBuilder methods?
public class StringBuilderExample
{
public static void main(String[] args)
{
StringBuilder sb = new StringBuilder(“Dilip pushpad”);
// append method
System.out.println(sb.append(” Indore”));
StringBuilder sb2 = new StringBuilder(“pushpad”);
//charAt method
System.out.println(sb2.charAt(4));
StringBuilder sb3 = new StringBuilder(“Pushpad Computer”);
//delete method
System.out.println(sb3.delete(3,5));
StringBuilder sb4 = new StringBuilder(“Dilip Sir”);
//deleteCharAt method
System.out.println(sb4.deleteCharAt(2));
StringBuilder sb5 = new StringBuilder(“Dilip Sir”);
StringBuilder sb6 = new StringBuilder(“Dilip Sir”);
//equal method
System.out.println(sb5.equals(sb6));
StringBuilder sb7 = new StringBuilder(“Dilip”);
//equal method
StringBuilder sb8 = sb7.append(“sir”);
System.out.println(sb7.equals(sb8));
StringBuilder sb9 = new StringBuilder(“dilipp Pushpad”);
//replace method
System.out.println(sb9.replace(5,7,”sir”));
StringBuilder sb10 = new StringBuilder(“dilip Pushpad”);
//indexOf method
System.out.println(sb10 .indexOf(“P”));
StringBuilder sb11 = new StringBuilder(“Pushpad”);
//lastindexOf method
System.out.println(sb11.lastIndexOf(“a”));
StringBuilder sb12 = new StringBuilder(“Pushpad”);
//insert method
System.out.println(sb12.insert(7,”Computer”));
StringBuilder sb13 = new StringBuilder(“Pushpad Sir”);
//reverse method
System.out.println(sb13.reverse());
StringBuilder sb14 = new StringBuilder(“Dilip Pushpad”);
//subSequence method
System.out.println(sb14.subSequence(3,7));
StringBuilder sb15 = new StringBuilder(“Pushpad”);
//substring method
System.out.println(sb15.substring(3));
StringBuilder sb16 = new StringBuilder(“Pushpad”);
System.out.println(sb16.capacity());
//Ensure capacity method
sb16.ensureCapacity(1000);
System.out.println(sb16.capacity());
StringBuilder sb17 = new StringBuilder(“Pushpad”);
//setCharAt method
sb17.setCharAt(4,’d’);
System.out.println(sb17);
StringBuilder sb18 = new StringBuilder(“Pushpad”);
//length method
sb18.setLength(5);
System.out.println(sb18);
StringBuilder sb19 = new StringBuilder(“dilip”);
sb19.ensureCapacity(100);
System.out.println(sb19.capacity());
//substring method
sb19.trimToSize();//remove extra memory
System.out.println(sb19.capacity());
}
}
output:-
Dilip pushpad Indore
p
Pusad Computer
Diip Sir
false
true
dilipsirPushpad
6
5
PushpadComputer
riS daphsuP
ip P
hpad
23
1000
Pushdad
Pushp
100
5
Q.5 defference between string , stringBuffer and stringBuilder ?
Work |
String |
StringBuffer |
StringBuilder |
storage |
Heap area and scp(string constant pool) |
Heap area |
Heap area |
object |
Immutable object |
Mutable object |
Mutable object |
memory |
If we change the value of string a lot of times it will allocate more memory. |
Consumes less memory |
Consumes less memory. |
Threads Safe |
Not thread safe |
All methods are synchronized & thus it is thread safe. |
Non – synchronized methods not – thread safe. |
performance |
Slow performance |
Fast as compared to string |
Fast as compared to stringBuffer |
use |
If data is not changing friqwently |
If data is changing friqwently |
If data is changing friqwently |
java swing
Q. What is Swing ?
Ans = String a Part of java extended Library. use of create desktop application.
import javax.swing.*;
AWT ( Abstract Window Toolkit )
Ans = 1. it was platform – dependent
2. it was heavy – weight
3. it has a limited set of components
Swing futures
Ans = 1. it is platform – independent
2. it is light – weight
3. it has more & powerful components.
Introduction To Course Components :-
- Basic Components
- Applications
- Event Listeners
- Layouts Managers
- Some Advace Components
- layers Of JFrame
- Look and Feel
- Projects
JFrame in Swing
- setVisible
- setDefaultCloseOperation( )
- setSize( )
- setLocation( )
- setBounds( )
- setIconImage( )
- setTitle( )
- setBackground( )
- setResizable( )
Q.1 Write a program to JFrame setVisible and setDefaultcloseOperation ?
Q.2 Write a program to JFrame setSize and setLocation ?
Q.3 Write a program to JFrame setBounds, setTitle , setIconImage , setBackground and setResizable ?
Q.4 Write a program to swing using constructor ?
Q.5 Write a program to swing extends JFrame ?
Q.6 Write a program create to new object jframe ?
JLabel in swing
- setText()
- setFont()
- How to use Image in JLable
- How to use both Image and Text in JLable
Q.1 Write a program JLabel ?
Q.2 Write a program JLable ?
Q.3 Write a program JLable replace Text ?
Q.4 Write a program JLable font ?
Q.5 Write a program JLable Image ?
Q.6 Write a program JLable Image and text ?
JTextField
- setText( )
- setFont( )
- setBackground( )
- setForeground( )
- setEditable( )
Q.1 Write a program to JTextField ?
JPasswordField create
- setEchorchar( )
- How to Hide / show Password
// JPasswordField Create
// 1. setEchoChar()
// 2. How to Hide / show Password
import javax.swing.*;
import java.awt.*;
class JPasswordField1{
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //close window running state
frame.setBounds(100,100,1000,500); //location and size
Container c=frame.getContentPane();
c.setLayout(null);
JPasswordField pass=new JPasswordField();
pass.setBounds(100,50,150,30);
c.add(pass);
pass.setText(“12345678”); //settext password
pass.setFont(new Font(“Arial”,Font.PLAIN,30));
pass.setEchoChar(‘*’); //print star
pass.setEchoChar((char)0);
}
}
JButton
- How to create
- setFont( )
- setText( )
- setForeground( )
- setBackground( )
- setCursor( )
- setEnabled( )
- setVisible( )
- how to use image in JButton
Q.1 write a program to create button?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(100,30);
btn.setLocation(100,100);
c.add(btn);
}
}
Q.2 write a program to increase button font size ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(150,40);
btn.setLocation(100,100);
c.add(btn);
Font font=new Font(“Arial”,Font.PLAIN,30);
btn.setFont(font);
}
}
Q.3 write a program to Change button text ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(200,40);
btn.setLocation(100,100);
c.add(btn);
Font font=new Font(“Arial”,Font.PLAIN,30);
btn.setFont(font);
btn.setText(“My Button”);
}
}
}
Q.4 write a program to button text color and background color changed ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(200,40);
btn.setLocation(100,100);
c.add(btn);
Font font=new Font(“Arial”,Font.PLAIN,30);
btn.setFont(font);
btn.setText(“My Button”);
btn.setForeground(Color.red); //text color
btn.setBackground(Color.yellow); //background color
}
}
Q.5 write a program to button charge hand curser ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(100,40);
btn.setLocation(100,100);
c.add(btn);
Cursor cur=new Cursor(Cursor.HAND_CURSOR);//hand cursor
btn.setCursor(cur);
}
}
Q.6 write a program to button change to corse curser ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(100,40);
btn.setLocation(100,100);
c.add(btn);
Cursor cur=new Cursor(Cursor.CROSSHAIR_CURSOR);//cross cursor
btn.setCursor(cur);
}
}
Q.7 write a program to button change to resize curser ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(100,40);
btn.setLocation(100,100);
c.add(btn);
Cursor cur=new Cursor(Cursor.W_RESIZE_CURSOR);//resize cursor
btn.setCursor(cur);
}
}
Q.8 write a program to button change to wait cursor ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(100,40);
btn.setLocation(100,100);
c.add(btn);
Cursor cur=new Cursor(Cursor.WAIT_CURSOR);//wait cursor
btn.setCursor(cur);
}
}
Q.9 write a program to button enable and disable ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(100,40);
btn.setLocation(100,100);
c.add(btn);
btn.setEnabled(false); // true = enable
}
}
Q.10 write a program to button show option ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
JButton btn=new JButton(“click me”);
btn.setSize(100,40);
btn.setLocation(100,100);
c.add(btn);
btn.setVisible(false); //true = show
}
}
Q.11 write a program to button image ?
import java.awt.Container;
import java.awt.Font;
import javax.swing.*;
import java.awt.*;
class jbutton1 {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // close window running state
frame.setBounds(100, 100, 1000, 500); // location and size
Container c = frame.getContentPane();
c.setLayout(null);
ImageIcon icon=new ImageIcon(“image/webside logo.png”);
JButton btn=new JButton(icon);
btn.setSize(icon.getIconWidth(),icon.getIconHeight()); //set image size button
btn.setLocation(100,100);
c.add(btn);
}
}
JButton Event
- ActionListener
- void actionPerformed( )
- addActionListener( )
Q.1 write a program to ActionListener ?
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
class Myframe extends JFrame implements ActionListener{
Container c;
JButton btn;
Myframe()
{
c=this.getContentPane();
c.setLayout(null);
btn=new JButton(“Clike me”);
btn.setBounds(100,100,100,50);
c.add(btn);
btn.addActionListener(this);
}
public void actionPerformed(ActionEvent e){
c.setBackground(Color.YELLOW);
}
}
class ActionDemo{
public static void main(String []args){
Myframe f=new Myframe();
f.setTitle(“Action Demo”);
f.setSize(700,500);
f.setLocation(100,100);
f.setVisible(true);
f.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE);
}
}
Q.2 write a program to ActionListener ?
Q.1 write a program create login form ? ( Project mini )
Q.2 write a program create login form and perform task ? (project mini 2)
Q.3 write a program to make simple calculator ? (mini Project)
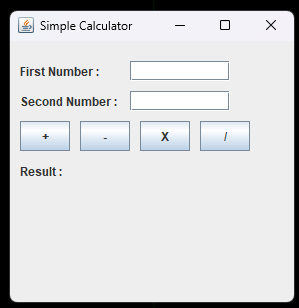
JTextArea
- How to create JTextArea
- setText(String )
- setFornt(Font)
- setEditable(boolean)
- setLineWrap(boolean)
Q.1 write a program to create JTextArea ?
import javax.swing.*;
Q.2 write a program to create JTextArea editing ?
JRadio Button
- How to create JRadio Button
- setFont (Font)
- setEnabled(boolean)
- ButtonGroup()
- How to create already selected radio buttons
- setSelected (boolean)
Q.1 Write a program to create JRadio Button ?

Q.2 Write a program to create JRadio Button group ?

Q.3 Write a program to create more than JRadio Button ?

JCheckBox
- How to Create
- setFont (Font)
- setEnabled (boolean)
- setSelected (boolean)
Q.1 Write a program to JCheckBox ?
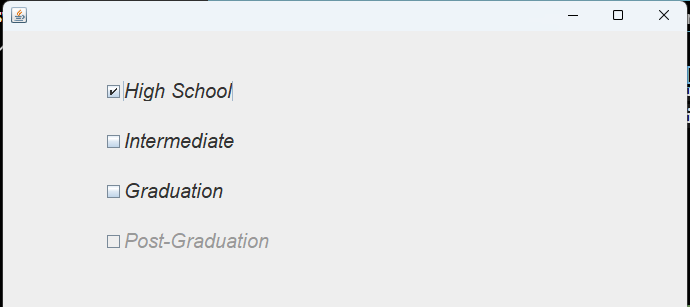
JComboBox
- How to create
- setEditable (boolean)
- setSelectedIndex(index)
- setSelectedItem(String)
- setFont(Font)
- getSelectedIndex()
- getSelectedItem()
- addItem()
- removeItem()
Q.1 w.a.p to create JComboBox ?
Q.2 w.a.p to create JComboBox ?
Q.3 W.A.P to create JComboBox ?
Q.4 write a program to create JBottun
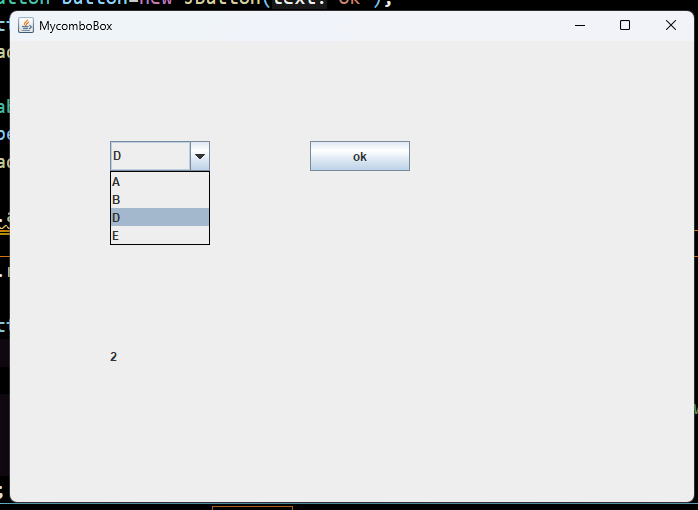
Q.5 write a program to Registration form ?

Q.6 write a program to Registration form ?
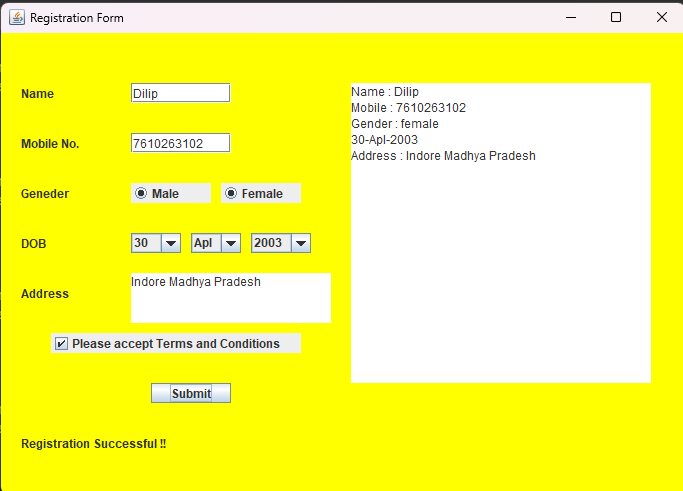
JAR Files :-
1. Jar Files are the executable files that can be run by double clicking on it.
2. A developer should not provide source code to its client/others so when he create a software he profide an executable file to its clients so that they can run it easily.
JMenuBar
- Parts of a menubar
- How to create a menubar in JFrame
Parts of a menubar :-
- MenuBar
- Menus
- MenuItems

Q.1 how to create file menu using by JMenuBar ?
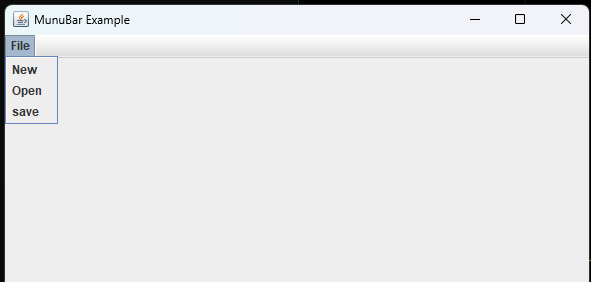
Q .2 write a program to create file and edit menu ?
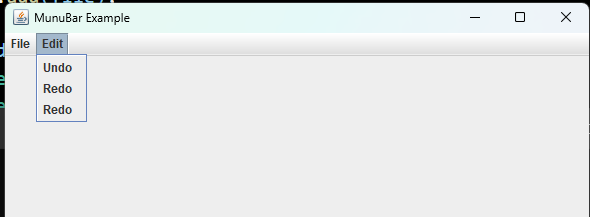
Q .3 write a program to create file sub menu ?
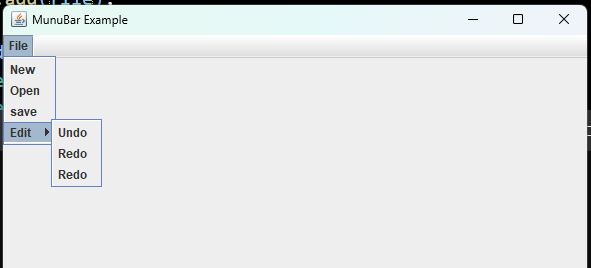
Q . what is Event Listeners ? full explain ?
Event Listeners :-
- Listeners
- Events
- Event Listeners
- Event Handling
Listeners
Anything that listens something is called a Listeners.
Events
All the change that we make when interacting with an application are called events.
example:- button clicked , Key Pressed , Mouse Pressed etc.
Every Componets has an state. and if we change the state of a component then it generates an event.
an Event is the change in the state of a componets.
Event Listeners
An Event Listener is a Listener that listens an Event.
Event Handling
To Handle the events is called event Handling.
and in java we handle the events with the help of event listeners.
So, To handle an event we need an Event Listener, and Handling those events is called Event Handling.
Different Types of Event Listeners ?
- ActionListener (ActionEvent)
- ItemListener (ItemEvent)
- KeyListener (KeyEvent)
- MourseListener (MourseEvent)
- MourseMotionListener (MourseMotionEvent)
- WindowListener (WindowEvent)
- FocusListener (FocusEvent)
- CaretListener (CaretEvent)
Q . write a program to create file and edit menu ?
JDBS stands for “Java Database Connectivity” . It is a API that is used to connect and excute the queries with the respective database.
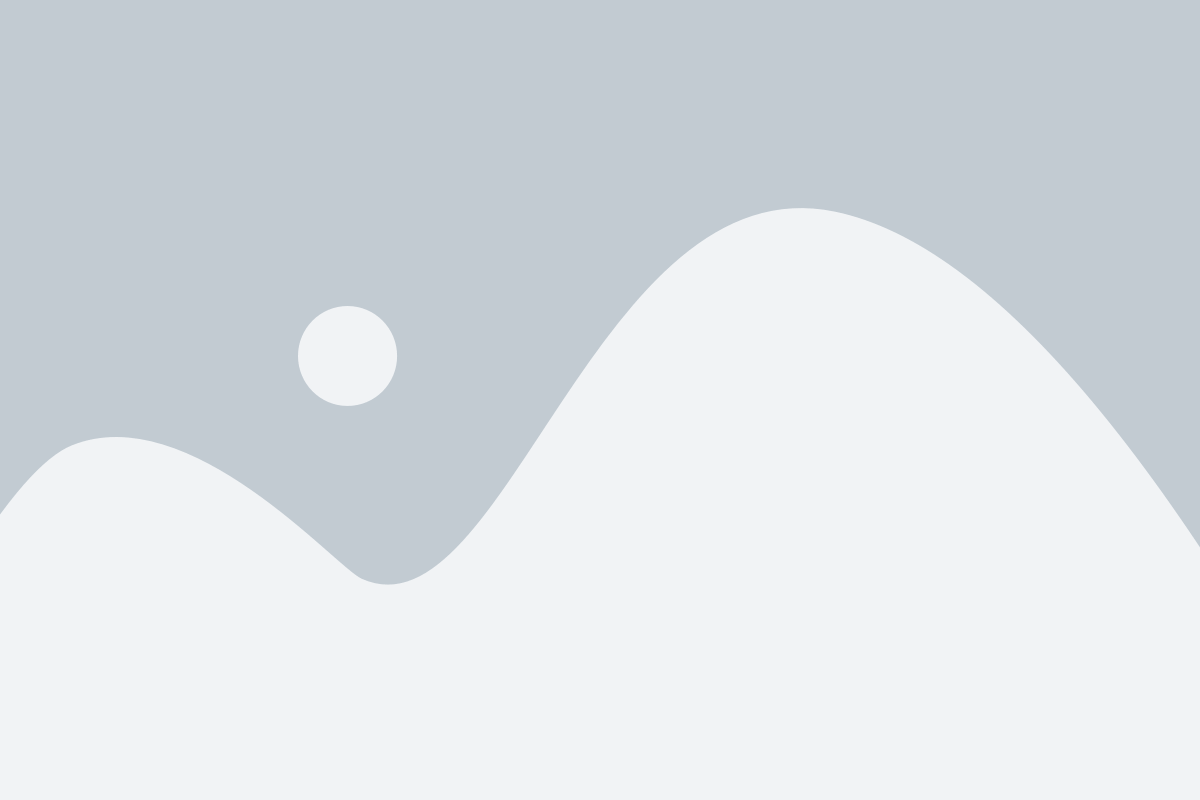
Q . write a program to create file and edit menu ?
JDBS stands for “Java Database Connectivity” . It is a API that is used to connect and excute the queries with the respective database.
JDBC ( Java Database Connectivity )
JDBS stands for “Java Database Connectivity” . It is a API that is used to connect and excute the queries with the respective database.
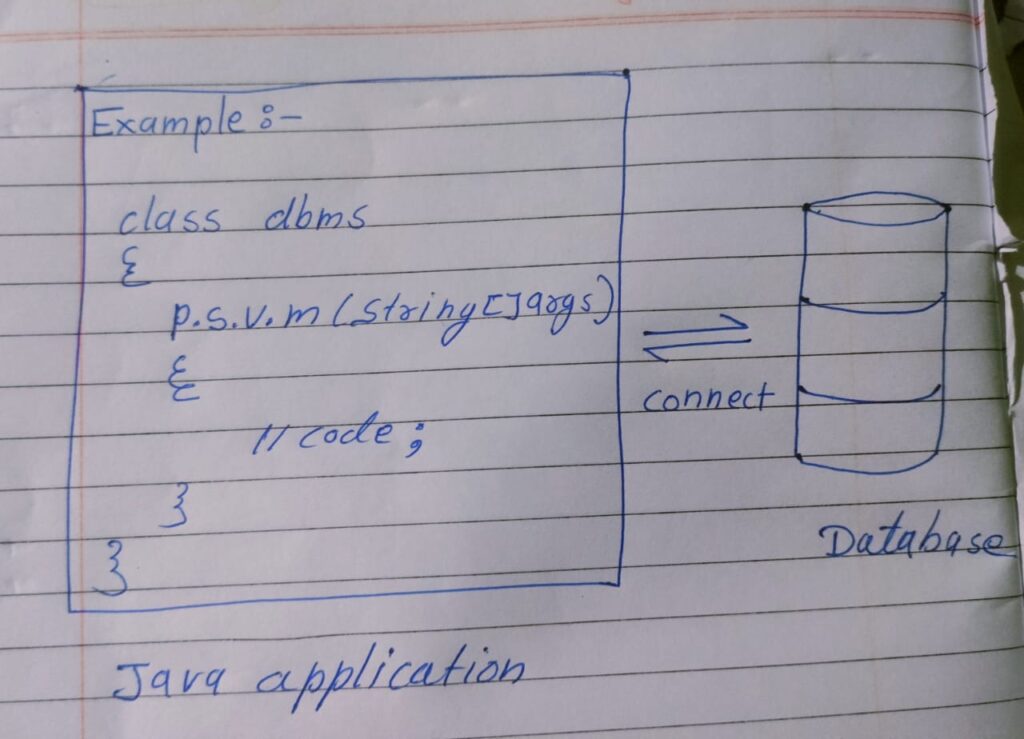
Note :- Requirement to perform JDBC
- JDK ( https://www.oracle.com/in/java/technologies/downloads/ )
- Eclipse ( IDE https://www.eclipse.org/downloads/ )
- Oracle database 11g ( https://www.oracle.com/database/technologies/xe-prior-release-downloads.html)
- .jar File
Oracle Database Connect
SQL> connect
Enter user-name: system
Enter password:
Step 1: Connect to database
1. class.forname()
2. connection interface
import java.sql.Connection;
import java.sql.DriverManager;
public class jdbs_connect
{
public static void main
(String[] args)
{
try
{
Class.forName(“oracle.jdbc.
driver.oracleDriver”);
Connection con=DriverManager.
getConnection(“jdbc:oracle:
thin:@localhost:1521
:xe”,“system”,“Dilip@1234”);
}
catch()
{
}
}
}
Step 2: Create Table in database:-
1. statement interface
2. execute Update();
import java.sql.Connection;
import java.sql.DriverManager;
public class jdbs_connect
{
public static void main
(String[] args)
{
try
{
Class.forName(“oracle.jdbc. driver.oracleDriver”);
Connection con=DriverManager.
getConnection(“jdbc:oracle:
thin:@localhost:1521:
xe”,“system”,“Dilip@1234”);
statement smt=con.
createStatement();
smt.executeUpdate
(“create table emp(eno number
,ename varchar(12),esal
number
“);
System.out.print(“Table
create successfully”);
con.close();
}
catch(Exception e)
{
System.out.print(e)
}
}
}
Step 3: insert record in database-
1. Prepared statement interface
Prepare statement( );
import java.io.BufferedReader;
import java.io.
InputStreamReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.
PreparedStatement;
public class JDBC_insert {
public static void main
(String[]args)
{
try
{
Class.forName(“oracle.jdbc.
driver.oracleDriver”);
Connection con=DriverManager.
getConnection(“jdbc:oracle:
thin:@localhost:1521:
xe”,“system”,“Dilip@1234”);
PreparedStatement psmt=con.
prepareStatement
(“insert into emp values
(?,?,?)”);
BufferedReader br=new
BufferedReader(new
InputStreamReader
(System,in));
while(true)
{
System.out.print(“Enter EMP \
Id: “);
int eno=Interger.parseInt
(br.readLine());
System.out.print(“Enter EMP Name: “);
String ename = br.readLine();
System.out.print(“Enter EMP Salary: “);
double sal=Double.parseDouble(br.readLine());
psmt.setInt(1,eno);
psmt.serString(3,ename);
psmt.setDouble(3,sal);
int count=psmt.executeUpdate();
if(count>0)
System.out.println(count+“record inserted”);
else
System.out.println(“No record inserted”);
System.out.println(“Do You Want to More Records[Yes/No]”);
String ch=br.readLine();
if(ch.equalsIgnoreCase(“no”));
break;
}
}
catch(Exception e)
{
System.out.print(e);
}
}
}
Step 4: Select record in database.
ResultSet interface
executeQuery();
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class DJBC_Select {
public static void main(String[] args) {
try
{
Class.forName(“oracle.jdbc.driver.oracleDriver”);
Connection con=DriverManager.getConnection(“jdbc:oracle:thin:@localhost:1521
:xe”,“system”,“Dilip@1234”);
statement smt=con.createStatement();
ResultSet rs=smt.executeQuery(“select * from emp”);
while(rs.next())
{
int eid=rs.getInt(1);
String ename=rs.getString(2);
double esal=rs.getDouble(3);
System.out.println(“Emp Id: “+eid);
System.out.println(“Emp Ename: “+ename);
System.out.println(“Emp Salary: “+esal);
System.out.println(“\n”);
}
}
catch(Exception e)
{
System.out.println(e);
}
}
}
Step 5: Update record in database -
Statement
execute Update( )
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
public class JDBC_Update {
public static void main(String[] args) {
String value; int eid; double esal;
try
{
Class.forName(“oracle.jdbc.driver.oracleDriver”);
Connection con=DriverManager.getConnection(“jdbc:oracle:thin:
@localhost:1521:xe”,“system”,“Dilip@1234”);
Statement smt=con.createStatement();
BufferedReader br=new BufferedReader(new InputStreamReader(System,in));
System.out.print(“Enter EMP Id: “);
value = br.readLine();
eid=Integer.parseInt(value);
System.out.print(“Enter Emp New Salary: “);
value = br.readLine();
esal=Double.parsoDouble(value);
int count=smt.executeUpdate(“update emp set esal= “+esal+“
where eno= “+eid);
if(count>0)
System.out.println(count+“rows updated”);
else
System.out.println(“No record found”);
}
catch(Exception e)
{
System.out.print(e);
}
}
}
Step 6: delete record in database -
statement = executeupdate( )
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
public class DJBC_Delete {
public static void main(String[] args) {
try
{
Class.forName(“oracle.jdbc.driver.oracleDriver”);
Connection con=DriverManager.getConnection(“jdbc:oracle:thin:@localhost:1521:xe”,“system”,“Dilip@1234”);
Statement smt=con.createStatement();
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
while(true)
{
System.out.print(“Enter Deletion EMP Id: “);
int eno=Integer.parseInt(br.readLine());
int count=smt.executeUpdate(“delete from emp where eno=”+eno);
if(count==1)
System.out.println(count+“rows deleted”);
else
System.out.println(“no record deleted”);
System.out.println(“Do You Want to More Records[Yes/No]”);
String ch=br.readLine();
if(ch.equalsIgnoreCase(“no”));
break;
}
}
catch(Exception e)
{
System.out.print(e);
}
}
}